Top Tools You Should Use for Optimizing Performance of ReactJS Apps
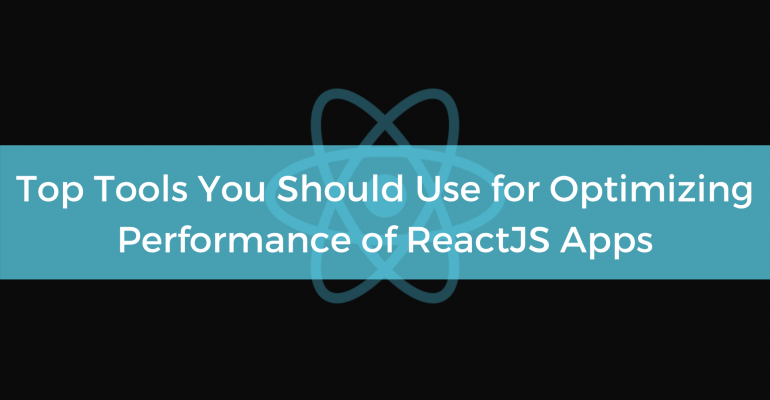
Many front-end developers choose React.Js for its excellent user interface designs. Though React.Js is a frontend JavaScript library, adding a React.Js library does not always yield fruitful results in your apps. It may lead to lags, bugs, and reusability problems as a developer you will never want to use mediocre codes over and over again.
In such case, optimizing React.Js can help you create faster and reliable apps. It can help developers manage all the coding issues. This guide will help you with how you can optimize React.js based app performance along with sharing few JavaScript best practices so as to experience fluid yet seamless experience.
How React.Js Works?
React.Js is a JavaScript-based library. It offers virtual DOM properties for developers to work with. With React.Js you can create an effective hierarchy of UI functions. It can help deliver excellent user experiences.
React.Js uses a tree-like structure for UI components. Each component has functions rendered to users. React keeps track of each user’s request and interaction with the system. If there are changes in the new UI, it compares them with the old UI. Later, it re-renders the UI with changes that are needed despite of the whole UI.
Unlike its counterparts like Angular.Js, React.Js doesn’t have the need for DOM manipulations. As these manipulations can slow things down, React provides faster development through the use of virtual DOMs. This is why majority startups, businesses and enterprises choose React.js framework over others.
React Developer Tools Extension: What’s that?
Before you start optimizing your React.Js apps, you need to know exactly, where do you need it? There are many tools available to help you with that. You can choose to install a React.Js Perf library for older versions. You can even use a why-did-you-update function. Then there is the chrome DevOps tool performance timeline for all your React-related analysis.
But, here we are discovering a dedicated chrome extension for React.Js developer tools. Once you install the extension, go to the React tab and select “highlight updates”. You can find the React tab in Chrome Dev Tools.
The tool helps to identify unnecessary re-rendering cycles. These are indicated through different color codes. You can track the updates in the User Interface. Developers can easily track changes ion re-rendering cycles with color codes. So, you can reduce the non-essential updates.
Now, that we know how React.Js works let’s get started with some quick and smart tips to optimize React.Js apps for high-performance.
Top Tools & Tricks You Can’t Afford To Miss Out For Optimizing React.Js App Performance
1.Using React.PureComponents:
- In Javascript, pure components help to modify the state of variables. These variables are the basis for programming functions. A React component is considered pure when it renders the same output on every state of variables. React.Js comes with a class of components. The class is better known as React.PureComponents.
- It controls the function automatically with shouldComponentUpdate. It performed a shallow comparison between different states and variables. If the states and props are the same from last rendering than they are pure components.
- It means that these components will compare and know whether the states and props are the same or not. If they are the same, it does not trigger the re-rendering of updates.
2. Immutable Data Structures:
- Data structures are always stored in a memory location. Changes to data structures or mutating data structures can affect your apps. You can hire React.js developers to induce immutability. It means that the basic structure of your app remains the same irrespective of changes in-app functions.
- Even changes due to the introduction of user-generated data should not undermine the original data structure of your app.
3. Using Elements:
React.Js allows the usage of JSX elements like values. So, you can use inline elements and constant elements. You can scale your values by reducing the calls to React.createClass . You can also use React inline elements to convert JSX components into the object literals.
To achieve the above functionality, you need to configure the following code in package.json.
"babel": {
"env": {
"production": {
"plugins": [
"transform-react-constant-elements",
"transform-react-inline-elements"
]
}
}
},
4. Using React.Lazy:
- Lazy loading is an amazing technique to optimize React apps. Take an example of an e-commerce app, where you want your users to see a payment option when requested by them. You can even render images as per a user request or a video when requested. It is a great way to speed up your apps.
- React. Lazy helps developers to load essential components of the app on user requests.It can reduce the loading time of apps. It uses a callback function parameter. Further the component file is loaded with an import() syntax.
You can use the following code for Lazy loading.
// MyComponent.js
class MyComponent extends Component{
render() {
return (<div>MyComponent</div>)
}
}
const MyComponent = React.lazy(()=>import('./MyComponent.js'))
function App() {
return (<div><MyComponent /></div>)
}
5. Using React.Suspense:
- Sometimes when a user requests for any specific component on the app like an image for example, there occurs a time lag. React. Suspense allows developers to represent feedback to users that there will be a time lag.
- As the screen may freeze during the lag, letting the user know of what’s coming can ensure user engagement. It allows the wrapping of lazy components into fallback content. Here, is an example of the code for React. Suspense.
6. Code-Splitting:
- There are much React.Js development companies1 that deliver high-end apps with bundled Javascript codes. However, it is a great method for smaller projects. It is not that great for a projects of bigger scale. For this, you can use a code-splitting technique.
- The feature offered by some React.Js development services can help minimize the bundled code. It helps developers to break these javascript bundled files into smaller chunks of codes.
7. ComponentDidUnmount():
- During the coding process there are several codes that are unused. When these codes are removed from the DOM, they are often stored as residual codes. These residual or unused codes can create a problem in React apps called the memory leak.
- Fortunately, React.Js comes with componentWillUnmount method. It removes any unwanted code when the related component is ditched. You can even use this technique to clean unnecessary subscriptions created for users. You can cancel network requests and other such non-essential codes.
Conclusion:
Debugging your apps without the help of a professional React.Js development company can be an error to regret. For delivering feature-rich customer experience, you need to put some effort. There are a lot of components, states, props, and other codes that can run on your app without the need. They can slow down your app and even force down a crash.
So, if you are looking to track all the updates, unnecessary codes, variables, and components, then choose the best optimization tools. These tools can certainly help your apps perform better and boost your business.
Want a professional consultation on React.Js optimization? Then feel free to share with us in the below comments section.